Graphics Processing
in OpenGL, C++
This is a course project which covers basic image processing, geometric transformations, geometric modeling of curves and surfaces, animation, 3-D viewing, visibility algorithms, shading, and ray tracing.
#3 Ray tracer
#3-3 Infinite Environment Lighting
This task is to implement a new type of light source: an infinite environment light.
[Image below: glass sphere with environment light]
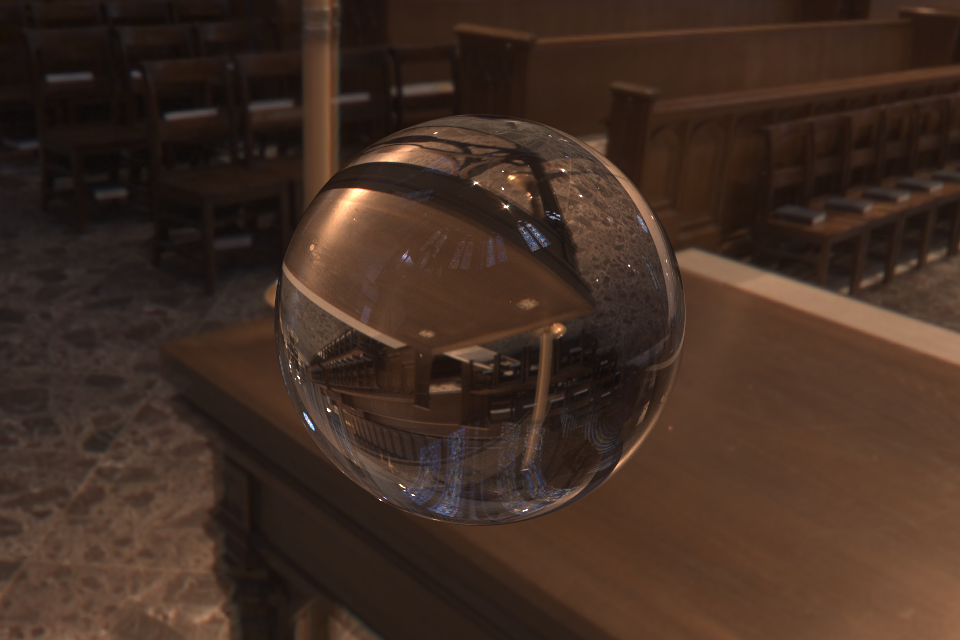
#3-2 Materials Adding
[Image below: spheres with perfect mirror and glass materials]
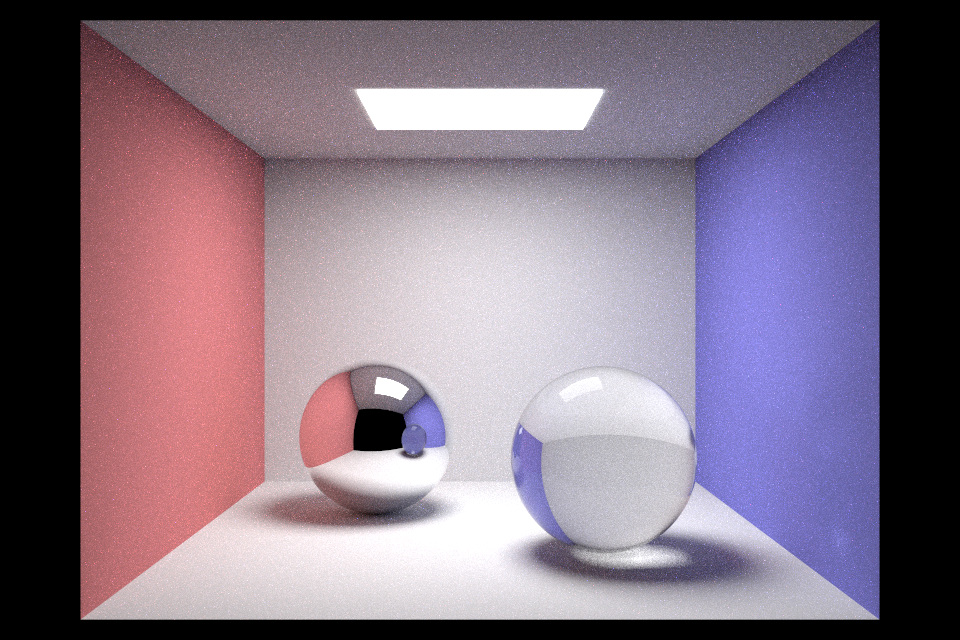
#3-1 Path Tracing
[Image below: lambertian sphere]

#3-0 BVH Building
[Image below: BVH building for the cow model]

#2 Mesh Editor
In this project I implemented a simple mesh editor that can manipulate and resample triangle meshes, which is a tool that allows you to load and edit basic COLLADA mesh files that are now used by many major modeling packages and real time graphics engines.
#2-3 Downsampling
In this task I implemented a method that simplifies a given model by applying quadric error simplification. This method was originally developed at CMU by Michael Garland and Paul Heckbert, in their paper Surface Simplification Using Quadric Error Metrics.
[Image below: Downsampling a beast model]
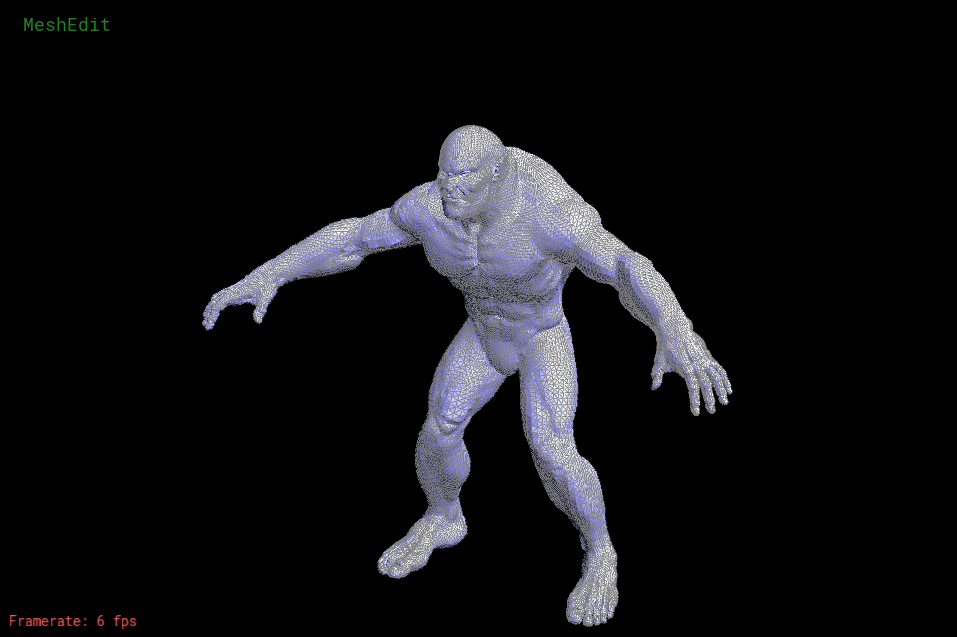
#2-2 Upsampling
In this task, I implemented an upsampling processing function that makes a low resolution image displayed at a higher resolution.
[Image below: Upsampling a low-sampled torus]
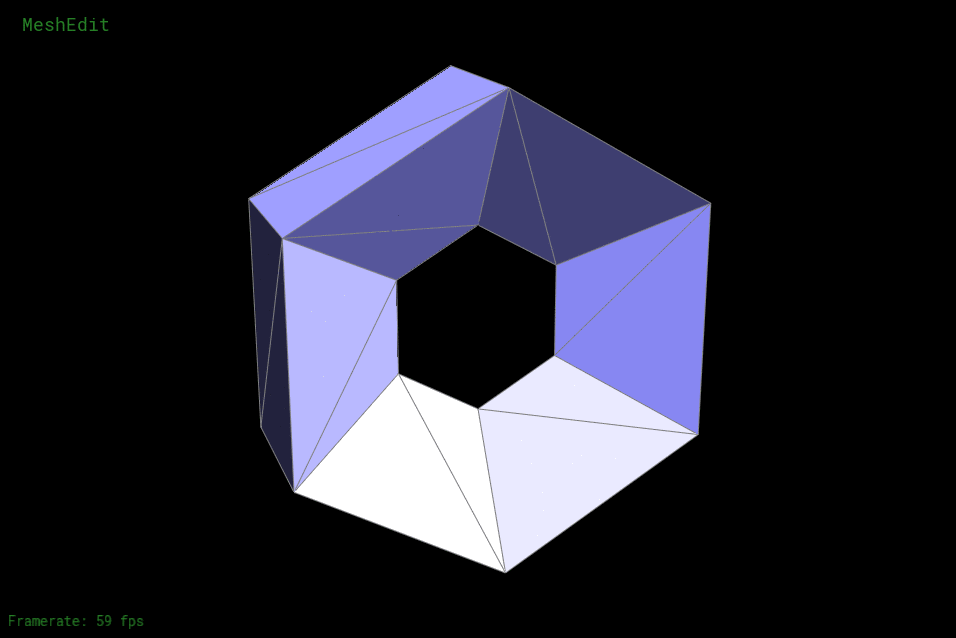
#2-1 Resampling
In this task, I implemented resampling via Isotropic Remising, which makes the mesh as "uniform" as possible.
[Image below: Resampling a quadball]
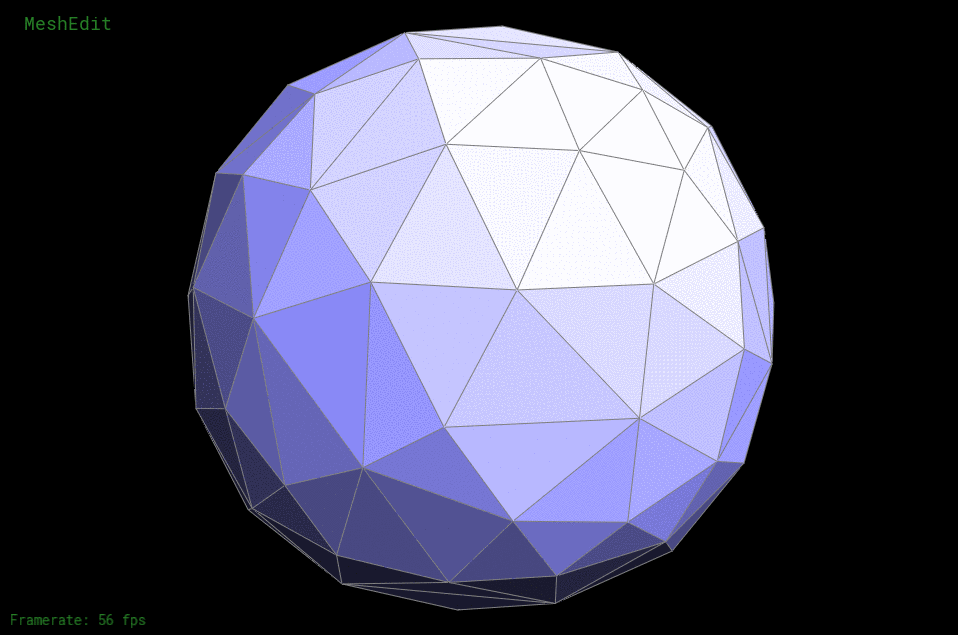
#2-0 Edge Flip / Split / Collapse
In this task I implemented a local remeshing operation that "flips" an edge, which:
#Handles corner cases.
#Performs efficiently.
#Is memory allocated clearly.
[Image below: Edge flipping, splitting, collapsing]
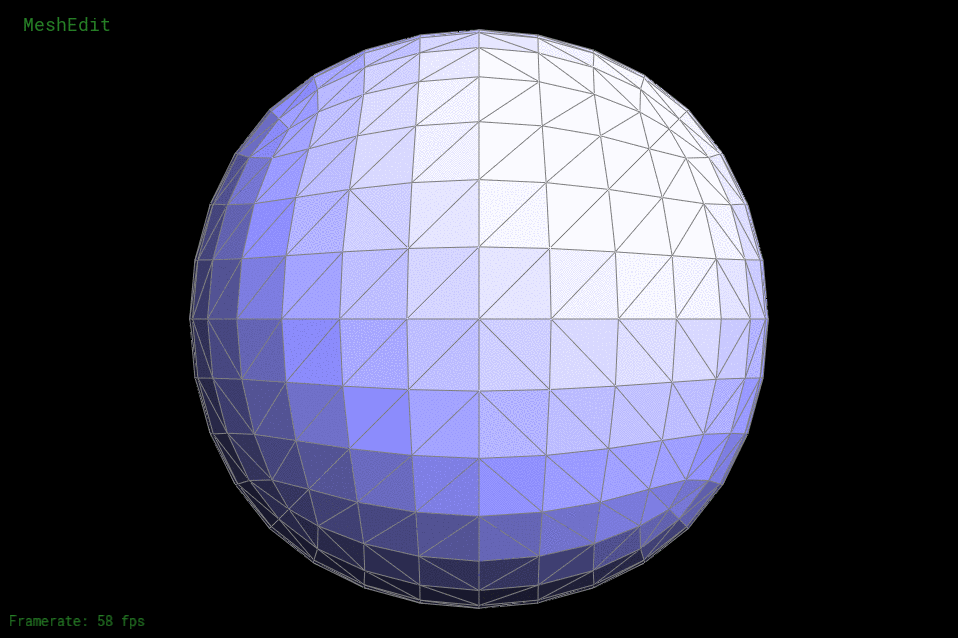
#1 SVG Drawer
In this project, I implemented a simple software rasterizer that draws points, lines, triangles, and bitmap images.
#1-4 Other implementations
Other implementations include:
#Modeling and Viewing Transforms.
#Anti-Aliasing Images with Bilinear, Trilinear filters and Mipmaps.
#Circle and Ellipse rasterizing.
#Alpha Compositing.
[Image below: Image drawn with functions implementation]
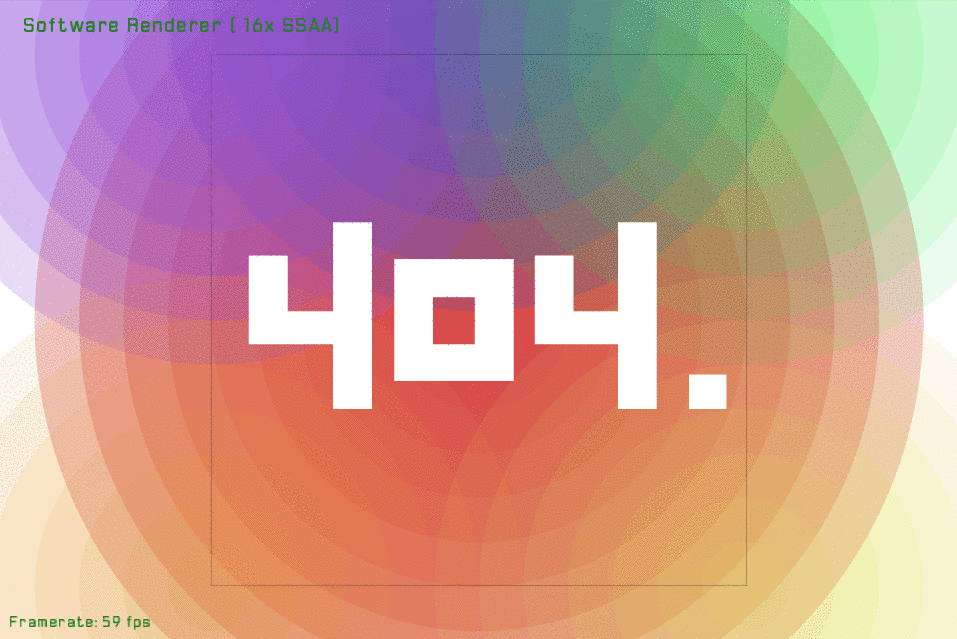
#1-3 Super-sampling
In this task, I extended my rasterizer to anti-alias triangle edges via supersampling in screen-space coordinates, which:
#Gives much smoother edges.
#Includes different supersampling rate in the viewer
[Image below: Increasing the supersampling rate]
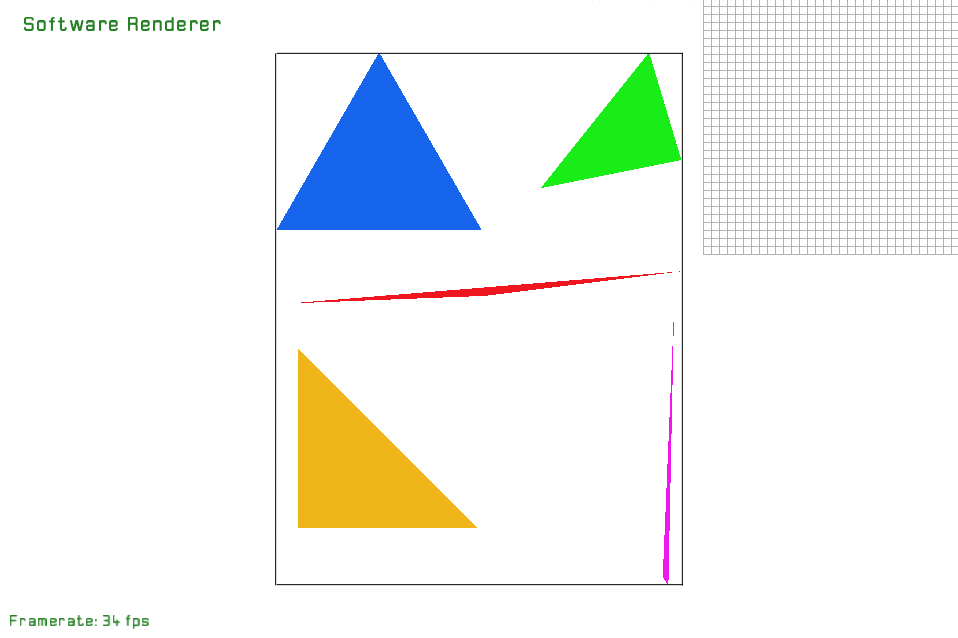
#1-2 Drawing Triangles
In this task I implemented rasterize_triangle which:
#Samples triangle coverage using the Point-in-triangle test.
#Respects the triangle "edge rules" to avoid "double counting".
#Uses an algorithm that is more work efficient than simply testing all samples on screen.
[Image below: Dragon drawing composed of triangles]
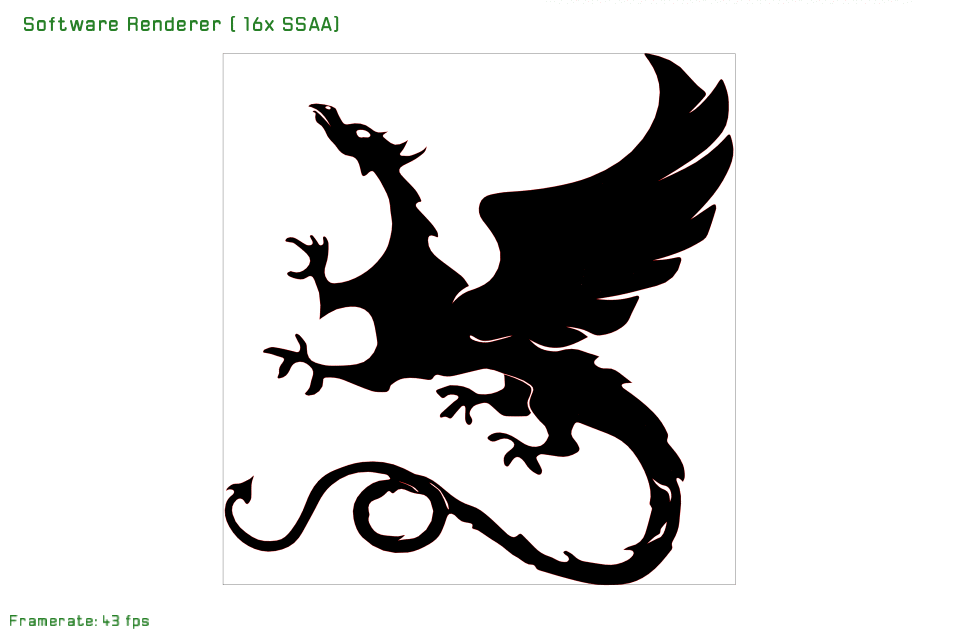
#1-1 Drawing Lines
In this task I implemented the function of rasterizing lines, which:
#Handles non-integer vertex coordinates.
#Handles lines of any slope.
#Performs work proportional to the length of the line.
#Includes Bresenham's algorithm and Xiaolin Wu's line algorithm.
[Image below: Line antialiasing implementation with Xiaolin Wu's algorithm]
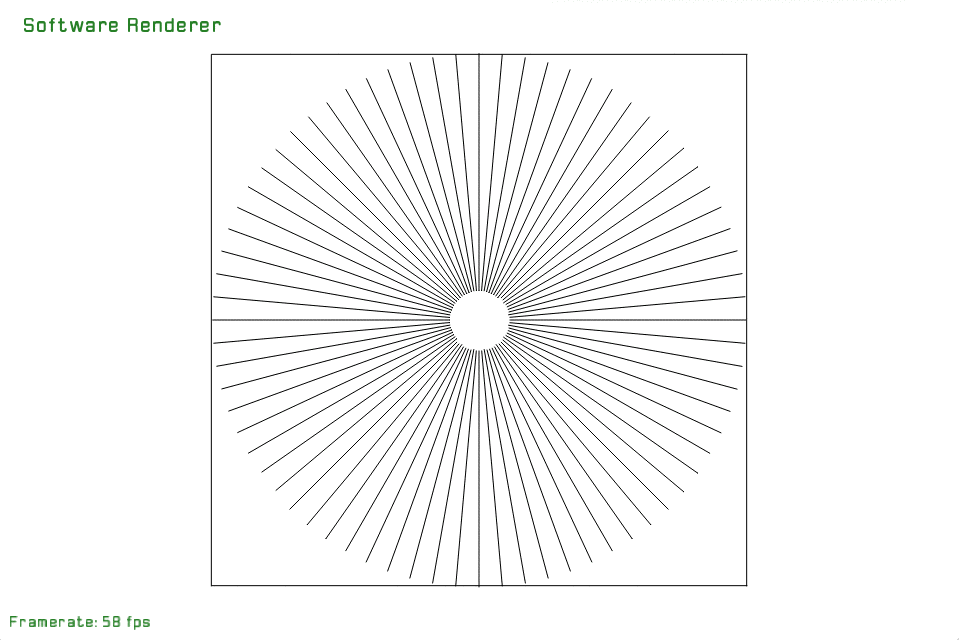